I recently needed to synchronize the ADC sample clock phase with a PWM output on the RPI Pico in uPython. Each upython assignment executes in several microseconds, that is just too slow in contrast the ADC’s sampling period of 2 us. The PWM register we need to set is located at:
$$ \text{ADDR}( \text{CH3_CSR} ) = \mathtt{0x40050000} + \mathtt{0x3C} $$
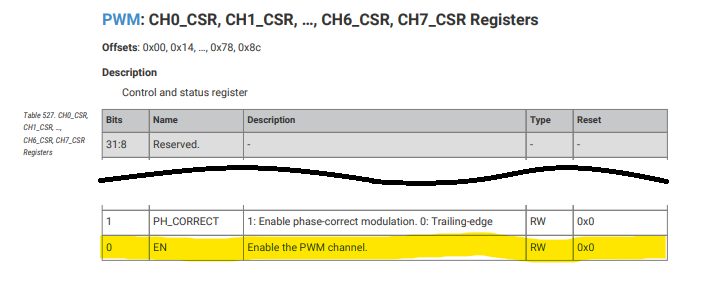
$$ \text{ADDR}( \text{ADC_CS} ) = \mathtt{0x4004c000} + \mathtt{0x00} $$
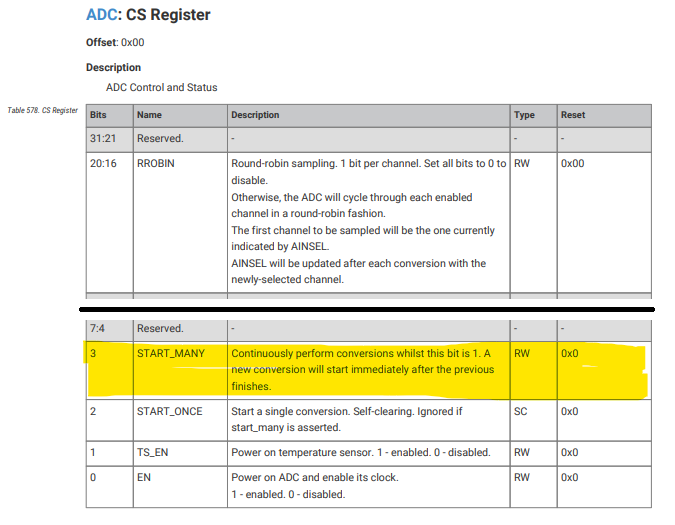
In uPython we will create an inline assembly function fastrun which takes a pointer to each of the registers described above.
@micropython.asm_thumb
def fastrun(r0,r1):
ldr(r2, [r0, 0]) # Load ADC_CS into r2
mov(r4,1)
lsl(r4,r4,3) # r4 = 1 << 3 = 0b1000
orr(r2,r4) # set bit in scratch reg
ldr(r3, [r1, 0]) # Load CH3_CSR into r3
mov(r4,1)
orr(r3,r4) # set EN bit
cpsid(1) # Disable Interrupts
str(r2, [r0, 0]) # write r2 to ADC_CS
str(r3, [r1, 0]) # write r3 to CH3_CSR
cpsie(1) # Enable Interrupts
treg = 0x40050000+0x3c
areg = 0x4004c000
# Enable PWM and ADC
fastrun(areg,treg)